You’ve mastered the basics of API documentation—great job! Now, it’s time to level up and tackle more complex API documentation challenges.
What Changes in Intermediate Level?
While beginner exercises helped you get comfortable with basic API structures, endpoints, and parameters, the intermediate level introduces:
- Multiple endpoints & real-world use cases (for example, Payments, Social Media, and Ride-sharing APIs).
- Authentication (API keys, OAuth, role-based access).
- Handling errors and failed API requests.
- Pagination, filtering, and query parameters.
- Rate limits, API security, and performance considerations.
This section prepares you for real-world API documentation work—where APIs are dynamic, complex, and must be crystal-clear for developers.
Let’s Dive Into Intermediate Challenges!
These exercises will help you build confidence by working on:
- Multi-endpoint documentation.
- Handling API authentication methods.
- Documenting error handling, rate limits, and best practices.
Each exercise builds on the last—so by the end of this section, you’ll have practical experience handling complex API documentation.
Exercise 1: Documenting a Payment Gateway API
Scenario: You are assigned to document the **Payment Gateway API**, which enables secure online payments.
Your Task: Write an API reference document covering:
- Overview of the API.
- Endpoints and HTTP methods.
- Authentication mechanism (OAuth, API keys).
- Request parameters in a structured table.
- An example API request (including cURL).
- A sample response from the API.
- Response parameters in a structured table.
- Handling failed transactions.
- Rate limits and API security best practices.
Provided API Code (Some details missing – you must fill them in):
Below is a sample API request and response:
API Request:
POST https://api.paymentgateway.com/transactions Headers: Authorization: Bearer {access_token} Content-Type: application/json Body: { "amount": 100.50, "currency": "USD", "payment_method": "credit_card", "card_number": "**** **** **** 4242", "expiry_date": "12/26", "cvv": "***", "merchant_id": "abc123" }
Sample Response:
{ "transaction_id": "txn_789456", "status": "Success", "amount": 100.50, "currency": "USD", "payment_method": "credit_card", "timestamp": "2025-02-17T10:00:00Z" }
Bonus Challenge: Document how a merchant should handle failed payments.
Solution to Exercise 1
1. Overview
The **Payment Gateway API** allows merchants to process secure online transactions using various payment methods such as credit cards and digital wallets.
2. Authentication
The API uses OAuth 2.0 authentication. To make a request, obtain an access token and include it in the `Authorization` header.
3. Endpoints and Methods
POST /transactions – Initiates a new payment transaction.
4. Request Parameters
Parameter | Type | Required | Default | Description |
---|---|---|---|---|
amount | Float | Yes | None | The transaction amount. |
currency | String | Yes | USD | Currency for the transaction. |
payment_method | String | Yes | None | Payment method (e.g., "credit_card"). |
merchant_id | String | Yes | None | Unique merchant identifier. |
5. Sample cURL Request
curl -X POST "https://api.paymentgateway.com/transactions" -H "Authorization: Bearer {access_token}" -H "Content-Type: application/json" -d '{ "amount": 100.50, "currency": "USD", "payment_method": "credit_card", "merchant_id": "abc123" }'
6. Error Handling
Common errors include:
Status Code | Message | Description |
---|---|---|
400 | Bad Request | Missing or invalid parameters. |
401 | Unauthorized | Invalid API token. |
402 | Payment Required | Payment declined by bank. |
Exercise 2: Documenting a Ride-Sharing API
Scenario: You are assigned to document the **Ride-Sharing API**, which allows users to book a ride, track its status, and cancel it if needed.
Your Task: Write an API reference document covering:
- Overview of the API.
- Endpoints and HTTP methods (Booking, Status, Cancellation).
- Authentication (OAuth tokens).
- Request parameters in a structured table.
- An example API request (including cURL).
- A sample response from the API.
- Response parameters in a structured table.
- Handling ride cancellations and unavailable drivers.
Provided API Code (Some details missing – you must fill them in):
Below is a sample API request and response:
API Request (Book a Ride):
POST https://api.ridesharing.com/book Headers: Authorization: Bearer {access_token} Content-Type: application/json Body: { "pickup_location": "123 Main St, New York", "dropoff_location": "456 Park Ave, New York", "vehicle_type": "Sedan", "payment_method": "credit_card" }
Sample Response:
{ "ride_id": "ride_987654", "status": "Confirmed", "estimated_fare": 20.50, "driver": { "name": "John Doe", "vehicle": "Toyota Camry", "license_plate": "XYZ-1234", "eta": "5 minutes" } }
Bonus Challenge: Document how users can track and cancel a ride.
Solution to Exercise 2
1. Overview
The **Ride-Sharing API** enables users to book rides, track their status, and cancel rides if necessary. It provides real-time updates on driver availability, estimated fare, and ride status.
2. Authentication
The API uses OAuth 2.0 authentication. Clients must request an access token and include it in the `Authorization` header.
3. Endpoints and Methods
- POST /book – Books a new ride.
- GET /status/{ride_id} – Retrieves the ride's current status.
- DELETE /cancel/{ride_id} – Cancels an ongoing ride.
4. Request Parameters (Booking)
Parameter | Type | Required | Default | Description |
---|---|---|---|---|
pickup_location | String | Yes | None | Pickup address for the ride. |
dropoff_location | String | Yes | None | Dropoff address for the ride. |
vehicle_type | String | No | Standard | Type of vehicle requested (Sedan, SUV, etc.). |
5. Sample cURL Request (Booking a Ride)
curl -X POST "https://api.ridesharing.com/book" -H "Authorization: Bearer {access_token}" -H "Content-Type: application/json" -d '{ "pickup_location": "123 Main St, New York", "dropoff_location": "456 Park Ave, New York", "vehicle_type": "Sedan", "payment_method": "credit_card" }'
6. Error Handling
Common errors include:
Status Code | Message | Description |
---|---|---|
401 | Unauthorized | Invalid or missing API token. |
404 | Not Found | Ride ID does not exist. |
409 | Driver Unavailable | No drivers are available at the moment. |
Exercise 3: Documenting a Social Media API
Scenario: You are assigned to document the **Social Media API**, which allows users to post content, retrieve a feed, and like posts.
Your Task: Write an API reference document covering:
- Overview of the API.
- Endpoints and HTTP methods (Post, Feed, Like).
- Authentication (OAuth tokens, user scopes).
- Request parameters in a structured table.
- An example API request (including cURL).
- A sample response from the API.
- Response parameters in a structured table.
- Handling rate limits and access permissions.
Provided API Code (Some details missing – you must fill them in):
Below is a sample API request and response:
API Request (Create a Post):
POST https://api.socialmedia.com/posts Headers: Authorization: Bearer {access_token} Content-Type: application/json Body: { "user_id": "user_12345", "content": "Exploring the new Social Media API!", "media_url": "https://example.com/image.jpg" }
Sample Response:
{ "post_id": "post_98765", "status": "Published", "user": { "id": "user_12345", "name": "John Doe", "profile_picture": "https://example.com/johndoe.jpg" }, "likes": 0, "timestamp": "2025-02-17T14:00:00Z" }
Bonus Challenge: Document how API users should handle rate limits and permissions.
Solution to Exercise 3
1. Overview
The **Social Media API** allows users to create posts, retrieve their feed, and interact with other users' content through likes.
2. Authentication
The API requires OAuth 2.0 authentication. Clients must request an access token and include it in the `Authorization` header.
3. Endpoints and Methods
- POST /posts – Creates a new post.
- GET /feed – Retrieves the latest posts from followed users.
- POST /posts/{post_id}/like – Likes a post.
4. Request Parameters (Creating a Post)
Parameter | Type | Required | Default | Description |
---|---|---|---|---|
user_id | String | Yes | None | Unique identifier of the user. |
content | String | Yes | None | Text content of the post. |
media_url | String | No | None | URL to an image or video. |
5. Sample cURL Request (Creating a Post)
curl -X POST "https://api.socialmedia.com/posts" -H "Authorization: Bearer {access_token}" -H "Content-Type: application/json" -d '{ "user_id": "user_12345", "content": "Exploring the new Social Media API!", "media_url": "https://example.com/image.jpg" }'
6. Error Handling
Common errors include:
Status Code | Message | Description |
---|---|---|
401 | Unauthorized | Invalid or missing API token. |
403 | Forbidden | User does not have permission to post. |
429 | Too Many Requests | Rate limit exceeded. |
Exercise 4: Documenting an E-Commerce API
Scenario: You are assigned to document the **E-Commerce API**, which allows users to browse products, place orders, and track order status.
Your Task: Write an API reference document covering:
- Overview of the API.
- Endpoints and HTTP methods (Product Search, Order Placement, Order Status).
- Authentication (API keys, role-based access control).
- Request parameters in a structured table.
- An example API request (including cURL).
- A sample response from the API.
- Response parameters in a structured table.
- Handling invalid orders and stock unavailability.
- Rate limits for API access.
Provided API Code (Some details missing – you must fill them in):
Below is a sample API request and response:
API Request (Place an Order):
POST https://api.ecommerce.com/orders Headers: Authorization: Bearer {api_key} Content-Type: application/json Body: { "customer_id": "cust_12345", "items": [ { "product_id": "prod_98765", "quantity": 2 } ], "payment_method": "credit_card", "shipping_address": "789 Commerce St, New York" }
Sample Response:
{ "order_id": "order_56789", "status": "Processing", "total_price": 50.00, "currency": "USD", "estimated_delivery": "2025-02-20", "items": [ { "product_id": "prod_98765", "name": "Wireless Headphones", "quantity": 2, "price_per_unit": 25.00 } ] }
Bonus Challenge: Document how users should handle stock unavailability.
Solution to Exercise 4
1. Overview
The **E-Commerce API** allows customers to browse products, place orders, and track order status in real-time.
2. Authentication
The API requires an API key for authentication. Customers and Admins have different access levels.
3. Endpoints and Methods
- GET /products – Retrieves a list of products.
- POST /orders – Places a new order.
- GET /orders/{order_id} – Retrieves order status.
4. Request Parameters (Placing an Order)
Parameter | Type | Required | Default | Description |
---|---|---|---|---|
customer_id | String | Yes | None | Unique identifier of the customer. |
items | Array | Yes | None | List of products and quantities. |
5. Sample cURL Request (Placing an Order)
curl -X POST "https://api.ecommerce.com/orders" -H "Authorization: Bearer {api_key}" -H "Content-Type: application/json" -d '{ "customer_id": "cust_12345", "items": [ { "product_id": "prod_98765", "quantity": 2 } ], "payment_method": "credit_card", "shipping_address": "789 Commerce St, New York" }'
6. Error Handling
Common errors include:
Status Code | Message | Description |
---|---|---|
401 | Unauthorized | Invalid or missing API key. |
404 | Product Not Found | The product ID is invalid. |
409 | Stock Unavailable | Product is out of stock. |
Exercise 5: Documenting a Weather Forecast API
Scenario: You are assigned to document the **Weather Forecast API**, which allows users to retrieve real-time weather updates and forecasts.
Your Task: Write an API reference document covering:
- Overview of the API.
- Endpoints and HTTP methods (Current Weather, Forecast, Alerts).
- Authentication (API key).
- Request parameters in a structured table.
- An example API request (including cURL).
- A sample response from the API.
- Response parameters in a structured table.
- Handling invalid locations and exceeded API limits.
- Rate limits and caching recommendations.
Provided API Code (Some details missing – you must fill them in):
Below is a sample API request and response:
API Request (Get Current Weather):
GET https://api.weatherforecast.com/current?location=New+York&apikey=your_api_key
Sample Response:
{ "location": { "city": "New York", "country": "US", "latitude": 40.7128, "longitude": -74.0060 }, "current_weather": { "temperature": 18, "humidity": 65, "wind_speed": 5.2, "condition": "Cloudy" }, "forecast": [ { "date": "2025-02-18", "temperature_min": 10, "temperature_max": 20, "condition": "Partly Cloudy" } ] }
Bonus Challenge: Document how API users should handle rate limits and caching best practices.
Solution to Exercise 5
1. Overview
The **Weather Forecast API** provides real-time weather updates, hourly and daily forecasts, and severe weather alerts for various locations worldwide.
2. Authentication
The API requires an API key for authentication. Each request must include the `apikey` parameter.
3. Endpoints and Methods
- GET /current – Retrieves current weather for a location.
- GET /forecast – Retrieves a 7-day weather forecast.
- GET /alerts – Retrieves active severe weather alerts.
4. Request Parameters (Current Weather)
Parameter | Type | Required | Default | Description |
---|---|---|---|---|
location | String | Yes | None | The name of the city (e.g., "New York"). |
apikey | String | Yes | None | API key for authentication. |
5. Sample cURL Request (Getting Current Weather)
curl -X GET "https://api.weatherforecast.com/current?location=New+York&apikey=your_api_key" -H "Accept: application/json"
6. Error Handling
Common errors include:
Status Code | Message | Description |
---|---|---|
401 | Unauthorized | Invalid or missing API key. |
404 | Location Not Found | Invalid city name or location not available. |
429 | Too Many Requests | API rate limit exceeded. |
Found value in the course? Your support fuels my work!
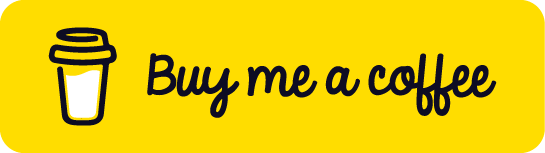
Course completed
Have an issue? Please provide specific feedback by reporting an issue.