Types of APIs - A Complete Overview
Discover the different types of APIs including REST, SOAP, GraphQL, WebSockets, JSON-RPC, XML-RPC, and gRPC. Learn how each API type works, their key features, and when to use them in your projects.
Table of Contents
Now that you know what we document and how we document, it’s time to explore the diverse world of APIs, and I’ll explain them in a way that’s easy to grasp.
We’ve six broad categories of APIs:
-
Web service APIs: These are like the superheroes of the internet. They help all sorts of websites and apps talk to each other. Some cool ones are REST, SOAP, GraphQL, and more.
-
Library-based APIs: Think of these as ready-made toolboxes for programmers. They come with handy tools (functions or classes) for doing specific jobs, like building stuff in a video game.
-
Class-based APIs (Object-Oriented): These are like special toolboxes that organize their tools in a very neat and organized way, just like how your toys might be sorted into different boxes.
-
Functions or routines in an OS: The operating system (the boss of your computer) shares some secret codes with programmers. These codes help programs use the computer’s special powers.
-
Object remoting APIs: Imagine if you could send messages to your friends using magic spells. Object remoting APIs are like those spells, making objects in different places talk to each other.
-
Hardware APIs: These are the keys to unlock the superpowers of your computer’s hardware, like the graphics card or the sound system.
In this tutorial, we are more interested in understanding Web service APIs. Why? Because 80-90% of the time, you are going to document Web service APIs only.
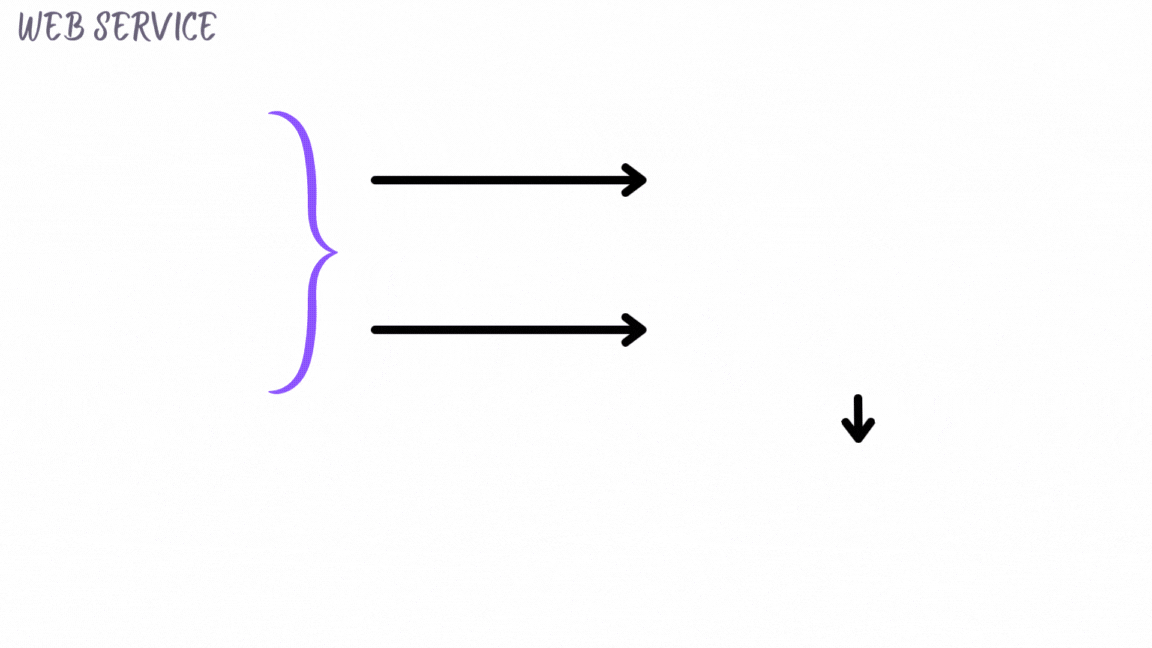
The Web service API encompasses:
- REST API
- SOAP API
- GraphQL
- WebSockets
- JSON-RPC
- XML-RPC
-
gRPC
Web Service API Types at a Glance
REST API
Most PopularSimple, stateless communication using HTTP methods and URLs
SOAP API
EnterpriseStrict, standardized protocol with built-in error handling and security
GraphQL
Rising StarQuery language that lets clients request exactly the data they need
WebSocket
Real-timePersistent connection allowing bi-directional real-time data flow
JSON-RPC/XML-RPC
SpecializedSimple remote procedure call protocols with minimal methods
gRPC
Performance-focusedHigh-performance RPC framework using Protocol Buffers and HTTP/2
REST API (Representational State Transfer)
So, first up, we have the REST API. Imagine it as the simplest way to design apps that talk to each other over the internet. It’s like going to a restaurant with a menu. You pick what you want (using simple commands like GET, POST, PUT, or DELETE), and the kitchen (the server) gets it ready and serves it to you. REST is known for being straightforward, scalable, and it works with pretty much anything on the web.
SOAP API (Simple Object Access Protocol)
Next, we’ve got the SOAP API. This one is a bit like sending letters, but with strict rules. Everything has to be in a specific order and format, just like how you need to write an address on an envelope in a particular way. SOAP is all about strong security and reliability, making sure your message gets to the right place.
GraphQL
Now, let’s talk about GraphQL. It’s like ordering a customised pizza. You get to choose exactly what toppings you want, and you won’t get anything else. With GraphQL, you request just the data you need in a single go. It’s super flexible and efficient, especially when you don’t want to waste time or data.
Try it yourself: GraphQL vs REST Data Fetching
With REST API: Need 3 separate requests
GET /api/user/123 // Returns all user fields, even if you need only name GET /api/user/123/posts // Returns all posts from user GET /api/user/123/followers // Returns all followers of user
With GraphQL: 1 request, only what you need
query { user(id: "123") { name posts { title date } followers { name } } }
WebSocket
Moving on to WebSockets. These are like having a phone call where you can talk and listen at the same time. It’s different from traditional mail (like sending letters) or email (where you send a message and wait for a response). WebSockets are great for real-time stuff like chatting and live updates.
JSON-RPC and XML-RPC
Now, let’s talk about JSON-RPC and XML-RPC. These are like asking a friend to do something specific for you, and your friend knows exactly what you want. It’s a way for one program to request a particular action from another program. These types are handy because they can work with different programming languages, making them a good choice for big, distributed systems.
gRPC
Last but not least, gRPC. Think of gRPC like sending a super efficient and structured letter with a built-in translator. It makes sure both the sender and the receiver understand each other perfectly. gRPC is known for high performance and strong typing, which means it’s fast and reliable.
Documentation Considerations for Different API Types
Documenting WebSocket APIs
Key challenges: Explaining event-based communication flows, documenting connection states and error handling.
Focus areas: Connection lifecycle, event types, message formats, and providing clear sequence diagrams.
Documenting GraphQL APIs
Key challenges: Explaining schema concepts, documenting nested relationships between types.
Focus areas: Interactive query explorers, schema documentation, mutation examples, and query variables usage.
Documenting SOAP APIs
Key challenges: Explaining complex XML structures and WSDL files to users.
Focus areas: Request/response XML examples, security implementations, error codes, and WSDL explanation.
Documenting gRPC APIs
Key challenges: Explaining Protocol Buffers and streaming concepts to users less familiar with them.
Focus areas: .proto file structure, service definitions, language-specific client examples, and stream handling patterns.
Intrigued by the fascinating world of APIs? Well, guess what? There’s more to explore in the next chapter, where we dive deeper into the superstar of APIs - REST APIs. Get ready for an exciting journey into its intricacies and applications. Stay tuned, and let’s unravel the secrets together!
Frequently Asked Questions About API Types
Get answers to common questions about different API types and when to use them.
REST API Fundamentals
REST (Representational State Transfer) is an architectural style for designing networked applications. A REST API works by using HTTP methods (GET, POST, PUT, DELETE, etc.) to perform operations on resources identified by URLs. Each resource is represented as a unique URL, and the HTTP method determines the action to perform on that resource. REST is stateless, meaning each request contains all the information needed to process it, and client-server communication occurs through standard HTTP requests and responses, typically using JSON or XML data formats.
The key principles of REST architecture include: 1) Statelessness - each request must contain all information needed, with no client context stored on the server; 2) Uniform Interface - resources are identified by URIs and manipulated through standard HTTP methods; 3) Client-Server separation - clients and servers evolve independently; 4) Cacheable - responses must define themselves as cacheable or non-cacheable; 5) Layered System - intermediary servers can improve scalability; and 6) Code on Demand (optional) - servers can temporarily extend client functionality by transferring executable code.
Essential documentation elements for REST APIs include: 1) Resource descriptions with clear URI patterns and examples; 2) HTTP methods supported for each resource; 3) Request parameters, headers, and body schemas with data types and constraints; 4) Response formats with status codes, headers, and body schemas; 5) Authentication and authorization requirements; 6) Error responses and handling; 7) Rate limiting policies; 8) Pagination mechanisms; 9) Working code examples in multiple languages; 10) Versioning information; and 11) Interactive API explorers when possible. Technical writers should focus on organizing this information logically by resource rather than by endpoint to improve usability.
SOAP API Fundamentals
SOAP (Simple Object Access Protocol) is a protocol for exchanging structured information using XML. Unlike REST which is an architectural style, SOAP is a formal protocol with strict standards. Key differences include: 1) SOAP uses XML exclusively while REST supports multiple formats like JSON; 2) SOAP typically operates over HTTP but can use other protocols like SMTP, while REST uses HTTP/HTTPS; 3) SOAP has built-in error handling and security features, while REST relies on the transport protocol; 4) SOAP is more rigid and formalized, while REST is flexible and lightweight; 5) SOAP has higher overhead due to XML parsing and strict messaging structure.
WSDL (Web Services Description Language) is an XML-based interface description language that describes the functionality offered by a SOAP web service. It provides a machine-readable description of how the service can be called, what parameters it expects, and what data structures it returns. UDDI (Universal Description, Discovery, and Integration) is a directory service where businesses can register and search for web services in a standardized way. It allows organizations to list their available SOAP services in a registry that can be discovered by others, functioning somewhat like a ‘phone book’ for web services. Together, they facilitate the discovery and consumption of SOAP web services in enterprise environments.
Technical writers documenting SOAP APIs should: 1) Explain the WSDL structure and how to interpret it; 2) Document each operation with its input and output messages, including all elements and types; 3) Provide detailed XML request and response examples for each operation; 4) Explain fault messages and error handling; 5) Document security requirements like WS-Security implementations; 6) Include code samples in multiple languages showing how to consume the SOAP service; 7) Explain any transport protocol considerations; 8) Document header elements and their purposes; 9) Create a glossary of domain-specific terms; and 10) Consider providing tools or guidance for generating client code from the WSDL. Given SOAP’s complexity, visual representations of message flows can be particularly valuable.
GraphQL API Fundamentals
GraphQL is a query language and runtime for APIs developed by Facebook. It works by having a single endpoint where clients can request exactly the data they need by specifying a query structure that mirrors the desired response structure. The server processes this query against its schema (which defines available types and fields) and returns only the requested data. Unlike REST, where the server determines the response structure, GraphQL gives clients control over what data they receive. GraphQL has three primary operation types: queries (for fetching data), mutations (for modifying data), and subscriptions (for real-time updates). The server resolves each field in the query independently, allowing for efficient and precise data fetching.
GraphQL schemas define the capabilities of an API by specifying the types of data that can be queried and how they relate to each other. A schema consists of: 1) Object Types - representing objects with fields (e.g., User, Product); 2) Scalar Types - primitive values like String, Int, Boolean, Float, and ID; 3) Query Type - defining entry points for read operations; 4) Mutation Type - defining entry points for write operations; 5) Subscription Type - defining entry points for real-time operations; 6) Input Types - special object types for arguments; 7) Enum Types - restricted sets of values; 8) Interface and Union Types - for polymorphic results; and 9) Custom Scalar Types for specialized data formats. The schema acts as a contract between client and server, enabling strong typing, validation, and documentation.
Documenting GraphQL APIs presents unique challenges including: 1) Explaining the conceptual shift from endpoint-based to schema-based thinking; 2) Documenting complex nested relationships between types; 3) Illustrating how to construct efficient queries to avoid performance issues; 4) Explaining pagination patterns and cursor-based approaches; 5) Documenting authentication that applies to the single endpoint; 6) Showing how error handling differs from REST APIs; 7) Explaining directives and their usage; and 8) Documenting schema evolution and deprecation practices. Technical writers should leverage introspection and tools like GraphiQL/GraphQL Playground to provide interactive documentation where users can explore the schema and test queries directly.
WebSocket API Fundamentals
WebSockets are a communication protocol providing full-duplex communication channels over a single TCP connection. They differ from HTTP-based APIs (like REST) in several ways: 1) Connection persistence - WebSockets maintain an open connection rather than creating a new connection for each request; 2) Bidirectional communication - both server and client can initiate messages at any time; 3) Real-time capabilities - messages are delivered immediately with minimal latency; 4) Different protocol - WebSockets use the ‘ws://’ or ‘wss://’ protocol rather than ‘http://’ or ‘https://’; 5) Message-based rather than request-response based; 6) Lightweight framing with minimal headers after the initial handshake. WebSockets are ideal for applications requiring real-time updates like chat, live dashboards, gaming, and collaborative editing.
Effective WebSocket API documentation should include: 1) Connection establishment details including any parameters required during handshake; 2) Authentication methods for secure connections; 3) Message formats with clear schemas for each message type; 4) Event types for both client-to-server and server-to-client communication; 5) Connection lifecycle including initialization, heartbeats, and graceful disconnection; 6) Error handling and reconnection strategies; 7) State management considerations; 8) Rate limiting or throttling information; 9) Sequence diagrams showing message flows for common scenarios; 10) Code examples showing connection, message handling, and error recovery; and 11) Any limitations or browser compatibility issues. Since WebSockets are event-driven, organizing documentation around events rather than endpoints is often more intuitive for users.
Common WebSocket API patterns include: 1) JSON-RPC over WebSockets - using RPC-style method calls with JSON serialization; 2) Event-based messaging - where messages are categorized by event types; 3) Publish/Subscribe - clients subscribe to topics and receive messages published to those topics; 4) Request/Response over WebSockets - maintaining the HTTP-style interaction but over a persistent connection; 5) Binary protocols - using binary formats like Protocol Buffers or MessagePack for efficiency; 6) Hybrid approaches - using REST for CRUD operations and WebSockets for real-time updates. Technical writers should document the chosen pattern clearly, as it significantly impacts how developers will interact with the API.
gRPC API Fundamentals
gRPC is a high-performance, open-source RPC (Remote Procedure Call) framework developed by Google. It uses HTTP/2 for transport and Protocol Buffers as its interface definition language. In gRPC, you define services that specify methods with their parameter and return types in .proto files. These are compiled into client and server code in various languages. When a client calls a remote method, the request is serialized using Protocol Buffers (a binary format), sent to the server, deserialized, and processed. The server then sends a response back using the same process. gRPC supports four types of service methods: unary RPC (single request/response), server streaming RPC, client streaming RPC, and bidirectional streaming RPC, enabling a wide range of communication patterns beyond what’s easily possible with REST.
Protocol Buffers (Protobuf) are Google’s language-neutral, platform-neutral, extensible mechanism for serializing structured data. They’re used in gRPC for several key reasons: 1) Compact binary format - resulting in smaller payloads (20-100% smaller than JSON) and faster transmission; 2) Schema definition - messages are strongly typed and defined in .proto files; 3) Code generation - automatically generates serialization/deserialization code in multiple languages; 4) Backward/forward compatibility - allows evolving APIs without breaking existing clients through carefully numbered fields; 5) Fast processing - more efficient to encode/decode than text-based formats like JSON or XML; 6) Structured data validation; 7) Built-in documentation through .proto files. Protocol Buffers are particularly valuable in high-throughput systems where performance and bandwidth efficiency are critical concerns.
Documenting gRPC APIs presents several unique challenges for technical writers: 1) Explaining Protocol Buffers syntax and concepts to users unfamiliar with IDL (Interface Definition Language); 2) Documenting streaming patterns which differ significantly from request-response models; 3) Explaining generated code usage across multiple programming languages; 4) Documenting interceptors and middleware concepts; 5) Explaining how to test gRPC services (as standard HTTP tools won’t work); 6) Documenting error handling with status codes and error details; 7) Explaining RPC concepts to developers familiar only with REST; 8) Documenting authentication implementation which differs from REST APIs. Effective documentation should include annotated .proto files, language-specific examples for all supported languages, and clear explanations of streaming concepts with sequence diagrams.
Documentation Best Practices for API Types
Documentation approaches vary significantly across API types: REST APIs typically require documenting individual endpoints with their HTTP methods, URL patterns, query parameters, request/response formats, and status codes, often using OpenAPI/Swagger specifications. GraphQL APIs center around documenting the schema (types, queries, mutations, and subscriptions) and can leverage introspection for self-documentation, with tools like GraphiQL providing interactive exploration. gRPC documentation focuses on service definitions and message types in .proto files, with emphasis on streaming patterns and generated client usage. While REST documentation organizes by endpoints, GraphQL by types, and gRPC by services, all benefit from authentication explanations, error handling details, and code examples. The technical writer’s approach should adapt to these fundamental structural differences while maintaining clarity and completeness.
When documenting API authentication across different types, technical writers should: 1) For REST APIs - explain header-based authentication (like API keys or Bearer tokens), query parameter options, and OAuth flows with clear request examples; 2) For GraphQL - document how authentication applies to the single endpoint, typically through headers, and how to handle authentication errors; 3) For SOAP - detail WS-Security implementations, SAML tokens, and XML signatures with examples; 4) For WebSockets - explain the initial handshake authentication and any session token requirements; 5) For gRPC - document interceptor-based authentication and metadata usage. For all types, include secure credential handling practices, token lifecycle management, permission scopes explanation, and environment-specific considerations (development vs. production). Visual diagrams of authentication flows are particularly valuable for OAuth and other complex mechanisms.
Technical writers should approach API versioning documentation differently based on the API type: For REST APIs, document the versioning strategy (URL path, query parameter, header, or content negotiation) with clear examples of accessing each version. For GraphQL, focus on schema evolution practices, including how deprecated fields are marked and for how long they’ll be supported. For SOAP, document different namespaces or endpoints for each major version and compatibility considerations. For gRPC, explain Protocol Buffer’s backward compatibility mechanisms, field numbering importance, and reserved fields. In all cases, clearly document the organization’s versioning policy, breaking vs. non-breaking changes, deprecation notices with timelines, migration guides between versions, and version-specific examples. Consider creating separate documentation sets for major versions while maintaining a clear navigation structure between them.
Key Takeaways
- Web service APIs like REST, SOAP, GraphQL, and WebSockets are the most commonly documented API types
- REST APIs are the most popular due to their simplicity, using HTTP methods and standard URLs
- SOAP APIs offer a more standardized approach with stricter protocols and XML formatting
- GraphQL allows clients to request exactly the data they need, reducing over-fetching
- WebSockets provide real-time, bi-directional communication through persistent connections
- gRPC is optimized for high-performance microservices communication
- Different API types serve different use cases and technical requirements
Test Your Knowledge
Learn More About API Types
Expand your knowledge with these carefully selected resources about different API types.